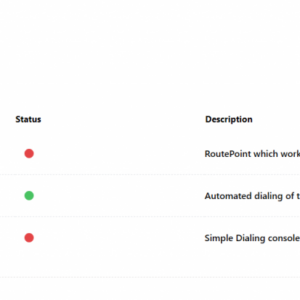
Create an outbound prompt campaign for easy automated calling.
Use the 3CX Call Control API to automate outbound call campaigns. Connect a list of phone numbers to an IVR, play an automated message, and transfer calls to different destinations based on IVR menu selections. Unlike traditional outbound dialing, this method provides flexibility in messaging, call routing and CRM or database integration. Read on to find out more and how to get started.
When to Use the Outbound Prompt Campaign Script
A flight cancellation is a perfect example. An airline can notify passengers via a recorded message and provide menu options to connect them to support.
This is a basic use case but it can be extended. You can build a custom IVR with DTMF input handling and audio stream control for outbound campaigns.
See the Official 3CX GitHub repository for more examples
Call Handling Setup & API Integration
Create a 3CX IVR, add it to Call Control API Access, and select it from the Extensions list.
Call Initiation
The client UI uses a simple text area to enter a comma-separated list of numbers.
const destinations = source
.split(',')
.map((num) => num.trim())
.filter(Boolean);
A queue structure processes calls one by one. Unanswered or busy calls can be queued for redialing.
destinations.forEach((destNumber) => this.callQueue.enqueue(destNumber));
Calling Logic
The function below retrieves the first number from the queue and starts processing.
public async makeCallsToDst() {
if (this.callQueue.isEmpty()) return;
const destNumber = this.callQueue.dequeue();
// …
Before dialing, the system checks the PBX connection and ensures the source extension is not in use.
if (!this.sourceDn || !this.externalApiSvc.connected) {
if (destNumber)
this.failedCalls.push({
callerId: destNumber,
reason: NO_SOURCE_OR_DISCONNECTED,
});
return;
}
const participants = this.getParticipantsOfDn(this.sourceDn);
if (participants && participants.size > 0) {
if (destNumber)
this.failedCalls.push({
callerId: destNumber,
reason: CAMPAIGN_SOURCE_BUSY,
});
return;
}
//…
Placing a Call
The call is placed using the first available device.
You can find the list of available devices for a specific DN within the State of The Call Control.
try {
const source = this.fullInfo?.callcontrol.get(this.sourceDn);
const device: DNDevice | undefined = source?.devices?.values().next().value;
if (!device?.device_id) {
throw new BadRequest('Devices not found');
}
const response = await this.externalApiSvc.makeCallFromDevice(
this.sourceDn,
encodeURIComponent(device.device_id),
destNumber,
);
//…
The makeCallFromDevice method uses this endpoint:
public makeCallFromDevice(source: string, deviceId: string, dest: string) {
const url="/callcontrol" + `/${source}` + '/devices' + `/${deviceId}` + '/makecall';
return this.fetch!.post(
url,
{
destination: dest,
},
{
headers: {
'Content-Type': 'application/json; charset=utf-8',
},
},
);
}
Error Handling
If the PBX accepts the request, the call ID is stored. Otherwise, an error is logged.
if (response.data.result?.id) {
this.incomingCallsParticipants.set(response.data.result.id, response.data.result);
} else {
this.failedCalls.push({
callerId: destNumber!,
reason: response?.data?.reasontext || UNKNOWN_CALL_ERROR,
});
}
//…
Errors between the application and PBX are handled here:
//...
} catch (error: unknown) {
if (axios.isAxiosError(error)) {
this.failedCalls.push({
callerId: destNumber!,
reason: error.response?.data.reasontext || UNKNOWN_CALL_ERROR,
});
} else {
this.failedCalls.push({
callerId: destNumber!,
reason: UNKNOWN_CALL_ERROR,
});
}
}
Participant Event Handling
A WebSocket connection tracks the IVR status, starts new calls, and manages participants.
You can find more details about the WebSocket event structure and other related aspects in this guide.
private wsEventHandler = (json: string) => {
try {
const wsEvent: WSEvent = JSON.parse(json);
if (!this.externalApiSvc.connected || !wsEvent?.event?.entity) {
return;
}
const { dn, type } = determineOperation(wsEvent.event.entity);
//...
When an update occurs, the application fetches and stores new data.
case EventType.Upset:
{
this.externalApiSvc
.requestUpdatedEntityFromWebhookEvent(wsEvent)
.then((res) => {
const data = res.data;
set(this.fullInfo, wsEvent.event.entity, data); // update local state
if (dn === this.sourceDn) {
if (type === PARTICIPANT_TYPE_UPDATE) {
/**
* handle here update of participants
*/
}
}
})
.catch((err) => {
if (axios.isAxiosError(err)) {
console.error(`AXIOS ERROR code: ${err.response?.status}`);
} else console.error('Unknown error', err);
});
}
break;
We can use this URL to request the updated entity and perform an incremental state update for our application (check DN Update Request):
public requestUpdatedEntityFromWebhookEvent(ws: WSEvent) {
return this.fetch.get(ws.event.entity);
}
When a participant is removed, the campaign continues.
case EventType.Remove: {
const removed = set(this.fullInfo, wsEvent.event.entity, undefined);
if (dn === this.sourceDn) { // update related to our campaign handler
if (type === PARTICIPANT_TYPE_UPDATE) {// update related to call participant
/**
* handle here removed participants
*/
if (removed?.id) {
//...
if (!participants || participants?.size < 1) { // Handler is free
this.makeCallsToDst(); // continue with campaign
}
}
}
}
}
We can use this event handler within the WebSocket event listener.
ws.on('message', (buffer) => {
const message = decoder.decode(buffer as Buffer);
wsEventHandler(message);
});
More Call Flow Scripts Available
We have a collection of call flow scripts on our website. Check them out and see how you can automate 3CX to fit your needs.
Source link
No Comment! Be the first one.